Claude 3: Function Calling and Tool Use
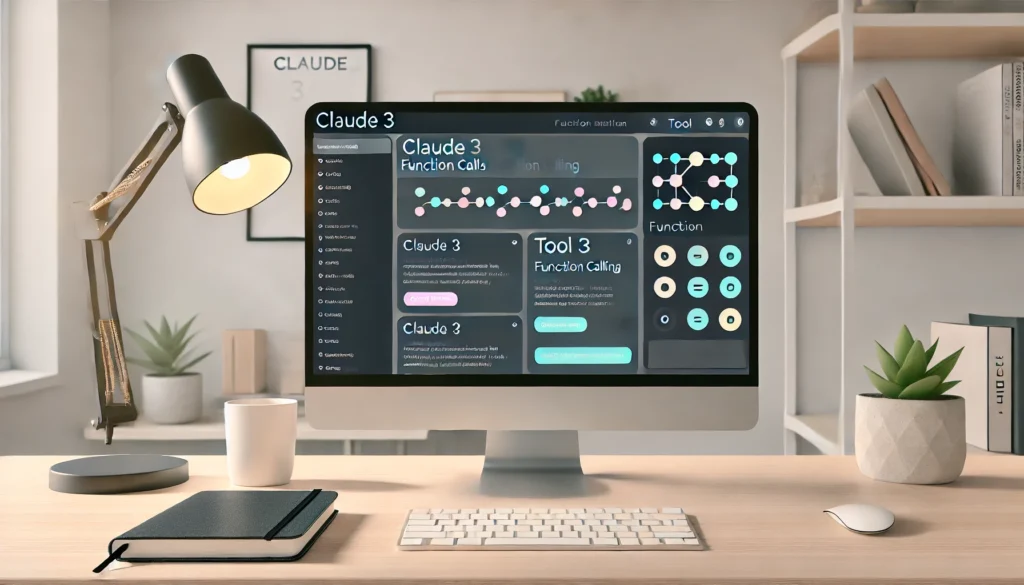
As artificial intelligence continues to change how we work and live, Claude 3 stands out with its exciting function calling and tool use features. Imagine having a virtual assistant who understands your questions and performs complex tasks for you—this is what Claude 3 offers.
Research shows that using AI tools can increase productivity by up to 40%, making these advanced features valuable to our daily routines. Function calling allows users to execute specific commands easily, making AI more helpful and user-friendly. Whether you’re managing data, automating tasks, or getting quick insights, Claude 3’s tools are designed to simplify these processes.
Let’s explore how Claude 3 is changing how we interact with AI, making our lives more efficient, and opening up new possibilities for using technology daily.
Introduction to Claude 3 Function Calling
As AI technology continues to evolve, the introduction of function calling in Claude 3 marks a significant leap forward in how large language models (LLMs) operate. This innovative feature allows users to seamlessly execute functions, making it easier to perform complex tasks like data analysis and programming.
Imagine automating repetitive tasks or running intricate queries without extensive coding knowledge—this is the power of function calling.
Claude 3 introduces an innovative approach to function calling that enhances usability and functionality. Function calling allows Claude 3 to execute specific commands and tasks directly based on user prompts. This means you can ask Claude to perform actions like retrieving data, generating reports, or even making calculations without sifting through complex code. This feature transforms Claude 3 into a more interactive tool, making it accessible for both tech-savvy users and those new to AI.
When compared with other large language models (LLMs), such as GPT-4 and Bard, Claude 3’s function-calling capabilities shine. While many LLMs provide basic interaction, Claude 3’s seamless integration of function calling allows for more dynamic and versatile applications.
Let us first understand how Claude’s function works.
How Claude Function Calling Works
Claude 3’s function calling capabilities provide a robust framework for users to interact with the model dynamically. At its core, function calling allows the model to execute predefined tasks or commands, streamlining various processes.
When you ask Claude to act—like retrieving data or running a calculation—it employs mechanisms that facilitate this interaction.
The function calling mechanism operates through a user-friendly interface. When you input a natural language request, Claude breaks it down to understand your intent. For example, if you ask, “What’s the weather in New York?” Claude interprets this as a request to fetch weather data, allowing it to trigger the relevant function.
Key Components of Function Calling
- Function Library
The function library houses a collection of predefined functions available to users. For instance, if you need to perform calculations, you can access functions like “calculate sum” or “find average.” This library simplifies the process, ensuring you have the right tools at your fingertips.
- Parameter System
The parameter system allows users to customize function execution. If you ask Claude to “calculate the total price for five items at $20 each,” the parameters (5 and $20) guide the function to deliver the desired output. This flexibility ensures that the functions can be tailored to specific user needs.
- Natural Language Processing (NLP) Algorithms
At the heart of Claude’s functionality is its advanced NLP algorithms. These algorithms help Claude accurately understand user requests. For example, if you say, “List my tasks for today,” the NLP component helps Claude identify “tasks” and “today” as key elements to extract relevant information.
Claude 3’s function calling mechanism enhances its ability to interact effectively with users by using a structured approach. The function library, parameter system, and NLP algorithms work together to provide efficient and relevant responses. This integration not only simplifies the user experience but also opens doors to diverse applications across various fields, from data retrieval to automated decision-making.
Now that we’ve covered the essential concepts behind function calling in Claude 3, it’s time to delve into the practical side of implementation.
Implementation Steps for Function Calling
This section will outline the key steps needed to implement function calling in Claude 3, ensuring a smooth setup and effective utilization.
1. Necessary Installations and Environmental Setup
Before using function calling with Claude 3, it’s essential to ensure that your development environment is properly set up. This includes installing necessary libraries and dependencies, such as the Claude SDK or relevant API clients.
Start by downloading the latest version from the official repository and following the installation instructions.
For example, if you’re using Python, you might run a command like pip install claude-sdk in your terminal. Setting up your environment correctly is crucial for smooth operation and to avoid common pitfalls.
2. Defining and Utilizing Functions for Data Retrieval
Once your environment is set up, the next step is defining the functions Claude will call. These functions should be tailored to meet specific data retrieval needs. For instance, you might create a function named get_weather_data(location) that fetches current weather conditions for a given location.
To define this function, you must outline the expected inputs (like location) and the output format (e.g., JSON containing temperature and humidity). Once defined, you can utilize this function within your application, allowing Claude to call it when necessary.
3. Constructing and Sending API Requests
The final step involves constructing and sending API requests to fetch data using the defined functions. This typically requires specifying the endpoint and including any necessary parameters.
For example, if your function get_weather_data calls an external weather API, you would format the request with the relevant URL and parameters.
Here’s a simplified example:
This line of code constructs a GET request to the weather API, sending the location as a parameter. After sending the request, you can process the returned data, integrating it seamlessly into your application.
Implementing function calling in Claude 3 involves three key steps: setting up your environment, defining functions for data retrieval, and constructing API requests. By following these steps, you can leverage the power of Claude 3 to enhance your applications and automate data handling efficiently. This structured approach not only streamlines the development process but also opens up numerous possibilities for integrating diverse functionalities.
Having established best practices for function calling, let’s explore how to leverage these techniques for integrating real-time data.
Using Function Calling for Real-Time Data
Integrating real-time data into applications can significantly enhance their functionality and user experience. Claude 3’s function calling feature makes this integration seamless and efficient. Using external APIs, developers can fetch live data directly within their applications, such as stock prices, weather updates, or social media feeds.
Examples of Real-Time Data Integration
For instance, imagine a financial app that displays real-time stock prices. By connecting to a stock market API, Claude 3 can pull current price data and present it instantly to users. Another example is a weather application that retrieves up-to-date weather information from an external API, allowing users to get live updates based on their location.
Step-by-Step Guide to Utilizing Claude for Fetching and Processing Data
- Set Up API Access: First, ensure you have access to the necessary external APIs. This often requires signing up for an API key to authenticate your requests.
- Define Functions: In Claude 3, define specific functions that outline how to retrieve data from the API. For example, a function could be designed to fetch stock prices based on a user’s input for the stock symbol.
- Make API Calls: Utilize the defined functions to send requests to the API. Claude 3 will process the request and fetch the data in real-time.
- Process and Display Data: Once the data is retrieved, use Claude 3 to process it as needed (e.g., formatting or calculations) and display it within your application.
Following these steps, you can effectively leverage Claude 3’s function-calling capabilities to create dynamic applications that provide real-time information, enhancing user engagement and satisfaction.
Now that we’ve explored how to use function calling for real-time data integration, it’s essential to focus on improving data processing capabilities.
Improving Data Processing with Function Calling
Function calling in Claude 3 offers powerful techniques to enhance data processing, especially when handling complex data formats like JSON. By implementing structured approaches, you can ensure that your applications work efficiently and maintain high data quality.
Techniques for Using JSON Schemas
JSON (JavaScript Object Notation) schemas define the structure of JSON data, making it easier to validate and process information. Adhering to a JSON schema can streamline data handling when using function calling. For instance, you can create a schema that specifies required fields for user profiles, such as name, email, and age. This helps in:
- Validation: Automatically check if incoming data adheres to the defined structure. For example, the system can return an error message if a user submits a profile without an email address, ensuring only valid data is processed.
- Documentation: A clear schema serves as documentation for your API, making it easier for developers to understand the expected data format and use it correctly.
Strategies for Maintaining Data Integrity and Consistency
Maintaining data integrity and consistency is crucial for reliable application performance. Here are some effective strategies:
- Data Validation: Beyond using JSON schemas, implement additional checks during data entry. For instance, if a user inputs a date, ensure it follows a specific format (e.g., YYYY-MM-DD). This reduces errors caused by invalid data.
- Error Handling: Develop robust mechanisms that provide informative feedback when data inconsistencies occur. For example, if a function encounters unexpected data types, it should gracefully handle the error and log it for review.
- Regular Audits: Schedule regular data audits to identify and rectify inconsistencies. This could involve checking for duplicates or ensuring all required fields are populated in your database.
- Version Control: If your data structure changes, implement version control for your API. This allows older applications to continue functioning while newer versions can utilize updated data formats.
By applying these techniques and strategies, you can significantly improve the efficiency of data processing with function calling in Claude 3. This not only enhances application performance but also builds trust with users through reliable data management.
Having discussed strategies for improving data processing with function calling, it’s crucial also to consider the best practices that can elevate your implementation.
Best Practices in Function Calling
Adhering to best practices can significantly enhance efficiency and reliability when working with function calling in Claude 3. Here’s a guide to help you define effective functions and optimize your API usage.
Recommendations for Efficient Function Definitions
- Keep Functions Focused: Each function should serve a specific purpose. For instance, if you’re fetching user data, create a function solely for that task. This modularity makes your code easier to read, maintain, and debug.
Example: A function named fetchUserData() should only retrieve user data, while another function, processUserData(), can handle any needed data transformations. - Use Meaningful Names: Choose clear and descriptive names for your functions. A name like calculateSalesTax() is much more informative than simply naming it calculate(). This practice improves code readability for anyone working on the project.
- Implement Default Parameters: Use default parameters in your functions to make them more flexible. For example, if your function has a parameter for a specific date but defaults to the current date, users can call it without specifying a date if they want the latest data.
- Document Your Functions: Document what each function does, the parameters it accepts, and the expected return values. This helps other developers (and your future self) understand the code without needing to decipher it.
Avoidance of Errors and Optimization of API Usage
- Implement Error Handling: Always anticipate potential errors when making API calls. Utilize try-catch blocks to handle exceptions gracefully. This way, your application can recover from errors without crashing, providing users a better experience.
Example: If an API call fails due to network issues, your code could retry the request several times before informing the user. - Rate Limiting Awareness: Be mindful of the API’s rate limits to avoid throttling or blocking. Implement logic to manage how frequently you make requests, ensuring you stay within the allowed limits.
- Caching Responses: To optimize performance and reduce API calls, consider caching responses for frequently requested data. If a function retrieves user information that doesn’t change often, store it locally to reduce load times and API usage.
- Batch Requests: When possible, batch multiple requests into a single API call. This reduces the number of network requests and can improve overall application efficiency.
By following these best practices for function calling, you can enhance your applications’ performance and reliability. Efficient function definitions and optimized API usage not only lead to cleaner code but also ensure a smoother user experience, setting your projects up for success.
Application in Document Processing
Here, we explore how function calling can revolutionize document processing by automating data extraction and management for a law firm.
Example Scenario of Automated Document Processing
Imagine a mid-sized law firm inundated with documents daily, from client contracts to legal briefs. Manually processing these documents can be time-consuming and prone to errors. The firm automates its document processing workflow using Claude 3’s function calling capabilities.
For instance, the firm creates a function that extracts key data points from contracts—like names, dates, and amounts—and formats them into a structured database. This function can be called whenever a new document is uploaded, ensuring that information is consistently captured and stored without manual intervention.
Role of Composio
Composio is a powerful tool that greatly improves document processing through several useful features. It automates document creation by using templates and standard formats. With its real-time collaboration capabilities, multiple users can work on documents simultaneously, making it easy to share feedback and make edits.
Composio also offers strong version control, allowing users to track changes and go back to earlier versions if needed. This is especially helpful for teams like legal firms and researchers.
Additionally, it can extract and analyze data from documents, making it easier for organizations to handle large amounts of information. The tool integrates smoothly with other applications, which helps create a more efficient workflow by allowing easy access to cloud storage.
Furthermore, Composio enhances searchability by indexing content, so users can quickly find specific information, which is especially useful in fast-paced settings like law offices. Overall, Composio is a valuable resource for businesses and organizations across various fields.
Conclusion
As we wrap up our exploration of function calling in AI, it’s clear that this feature holds significant promise for enhancing task automation and data processing.
Function calling in AI offers tremendous potential for automating tasks and improving efficiency across various applications. Allowing seamless integration of external functions enhances the capabilities of AI systems, enabling them to process data more effectively and respond in real-time. As technology advances, we can expect further innovations in function calling, paving the way for even more sophisticated applications.
Experience seamless function calling and tool integration that streamline your workflow and boost productivity. Whether you’re generating reports, pulling in data, or automating tasks, Composio makes it easier than ever.
Don’t miss out on transforming your document management—try Composio today and see the difference that Claude 3 can make!