
Skills that evolve for
your Agents
More than just integrations, 3000+ tools that
can adapt — turning automation into intuition.
More than just integrations, 3000+ tools that can
adapt — turning automation into intuition.
Skills that evolve for
your Agents
More than just integrations, 3000+ tools that
can adapt — turning automation into intuition.
Used by Agents from
Used by Agents from
Muscle Memory
Muscle Memory
for Intelligence
for Intelligence
successful calls
7,000,000+
successful calls
In a world with countries of geniuses in
datacenters, we believe the most important
thing is for them to be able to take complex
actions and learn from them in realtime — to
build intuitions, to build skills, they can plug
& play to create real economic value.
We are creating that layer that lets
intelligence build intuition.
Muscle Memory
for Intelligence
successful calls
7,000,000+
successful calls
In a world with countries of geniuses in
datacenters, we believe the most important
thing is for them to be able to take complex
actions and learn from them in realtime — to
build intuitions, to build skills, they can plug
& play to create real economic value.
We are creating that layer that lets
intelligence build intuition.


Building agents that take action is hard
building integrations
optimising JSON schema for agents
scaling to millions of tools execution
managing auth and permissions for tools
Composio erases that drag in one call


Use an
AI Agent
to detect bugs in Slack, auto-log them
to GitHub and Notion
Product Manager

Use an
AI Agent
to detect bugs in Slack, auto-log them
to GitHub and Notion
Product Manager
Tools
Onboard and assign issues
Detect bugs and
auto-log them
LLM
Implement Slack, GitHub, and Notion APIs
# ---- GitHub ----
def create_github_issue(repo, title, body, token):
url = f"https://api.github.com/repos/{repo}/issues"
headers = {"Authorization": f"token {token}"}
data = {"title": title, "body": body}
return requests.post(url, json=data, headers=headers)
# ---- Notion ----
def log_to_notion(database_id, title, github_url, notion_token):
url = "https://api.notion.com/v1/pages"
headers = {
"Authorization": f"Bearer {notion_token}",
"Content-Type": "application/json",
"Notion-Version": "2022-06-28"
# ---- Slack ----
def reply_in_slack(channel, thread_ts, text, slack_token):
url = "https://slack.com/api/chat.postMessage"
headers = {"Authorization": f"Bearer {slack_token}"}
data = {
"channel": channel,
"thread_ts": thread_ts,
"text": text
}
return requests.post(url, json=data, headers=headers)
1
Manage 3 Different OAuth Flows (Slack,
GitHub, Notion)
# Redirect user to GitHub's OAuth URL
github_oauth_url = f"https://github.com/login/oauth/authorize?client_id={CLIENT_ID}&scope=repo"
# User is redirected back with a ?code=xyz
# Exchange code for access token
def exchange_github_code_for_token(code):
response = requests.post(
"https://github.com/login/oauth/access_token",
headers={"Accept": "application/json"},
data={
"client_id": CLIENT_ID,
"client_secret": CLIENT_SECRET,
"code": code,
},
)
return response.json().get("access_token")
2
Create a Slack Event Subscription and Webhook Endpoint
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route("/slack/events", methods=["POST"])
def handle_event():
data = request.json
# Slack verification challenge
if data.get("type") == "url_verification":
return jsonify({"challenge": data["challenge"]})
event = data.get("event", {})
if event.get("type") == "message"
3










hrs
without Composio
with Composio



Turn bugs on Discord thread into Github issues and sync with Calendar events.
Toolkits required



bug details extracted
issues created
'bug bash' event added
notifications sent
time taken →
time taken →
time taken →
time taken →
Composio
An all in one seamless layer that lets LLMs and agents reliably interact with tools in the real world.
Authentication
Manage triggers
Set tools and actions
Use multi-agents
LLM
Composio listens to your LLM’s function or tool calls, handles authentication, maps the call to a real-world API, and executes it reliably.


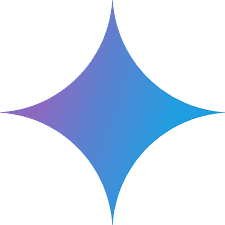

AI Agent
Connect with over 25 agentic frameworks, allowing AI agents to autonomously plan, coordinate, and execute actions across tools while your agent focuses on planning and execution is handled seamlessly.





Tools
Onboard and assign issues
Detect bugs and
auto-log them
AI Agent
Lang Chain
Lang Graph
AutoGen
Vercel
CrewAI
Lang Graph
Crew AI
Vercel
Lang Chain
Auto Gen
LLM
Open AI
Claude
Gemini
Grok
Open AI
Claude
Gemini
Grok


Turn bugs on Discord thread into Github issues and sync with Calendar events.
Toolkits required



bug details extracted
issues created
'bug bash' events added
notifications sent
time taken →
time taken →
time taken →
time taken →
Composio
An all in one seamless layer that lets LLMs and agents reliably interact with tools in the real world.
Authentication
Manage triggers
Set tools and actions
Use multi-agents
LLM
Composio listens to your LLM’s function or tool calls, handles authentication, maps the call to a real-world API, and executes it reliably.


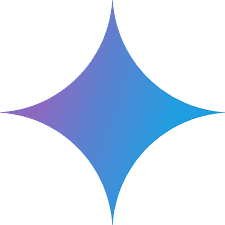

AI Agent
Connect with over 25 agentic frameworks, allowing AI agents to autonomously plan, coordinate, and execute actions across tools while your agent focuses on planning and execution is handled seamlessly.





Tools
Onboard and assign issues
Detect bugs and
auto-log them
AI Agent
Lang Chain
Lang Graph
AutoGen
Vercel
CrewAI
Lang Graph
Crew AI
Vercel
Lang Chain
Auto Gen
LLM
Open AI
Claude
Gemini
Grok
Open AI
Claude
Gemini
Grok


Turn bugs on Discord thread into Github issues and sync with Calendar events.
Toolkits required



bug details extracted
issues created
'bug bash' events added
notifications sent
time taken →
time taken →
time taken →
time taken →
Composio
An all in one seamless layer that lets LLMs and agents reliably interact with tools in the real world.
Authentication
Manage triggers
Set tools and actions
Use multi-agents
LLM
Composio listens to your LLM’s function or tool calls, handles authentication, maps the call to a real-world API, and executes it reliably.


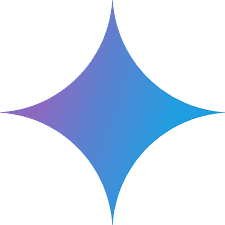

AI Agent
Connect with over 25 agentic frameworks, allowing AI agents to autonomously plan, coordinate, and execute actions across tools while your agent focuses on planning and execution is handled seamlessly.








Turn bugs on Discord thread into Github issues and sync with Calendar events.
Toolkits required



bug details extracted
issues created
'bug bash' event added
notifications sent
time taken →
time taken →
time taken →
time taken →
Composio
An all in one seamless layer that lets LLMs and agents reliably interact with tools in the real world.
Authentication
Manage triggers
Set tools and actions
Use multi-agents
LLM
Composio listens to your LLM’s function or tool calls, handles authentication, maps the call to a real-world API, and executes it reliably.


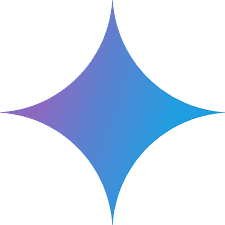

AI Agent
Connect with over 25 agentic frameworks, allowing AI agents to autonomously plan, coordinate, and execute actions across tools while your agent focuses on planning and execution is handled seamlessly.





Tools
Onboard and assign issues
Detect bugs and
auto-log them
AI Agent
Lang Chain
Lang Graph
AutoGen
Vercel
CrewAI
Lang Graph
Crew AI
Vercel
Lang Chain
Auto Gen
LLM
Open AI
Claude
Gemini
Grok
Open AI
Claude
Gemini
Grok


Turn bugs on Discord thread into Github issues and sync with Calendar events.
Toolkits required



bug details extracted
issues created
'bug bash' events added
notifications sent
time taken →
time taken →
time taken →
time taken →
Composio
An all in one seamless layer that lets LLMs and agents reliably interact with tools in the real world.
Authentication
Manage triggers
Set tools and actions
Use multi-agents
LLM
Composio listens to your LLM’s function or tool calls, handles authentication, maps the call to a real-world API, and executes it reliably.


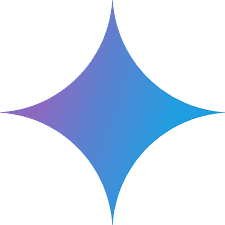

AI Agent
Connect with over 25 agentic frameworks, allowing AI agents to autonomously plan, coordinate, and execute actions across tools while your agent focuses on planning and execution is handled seamlessly.





00
0.5
1
2
3
4
5
6
7
8
9
00
10
11
12
13
14
00
16
17
18
00
Built
for Agents
Built
for Agents
*
*
Our easy-to-use APIs make it effortless to ship agents.
*Developers welcome
Our easy-to-use APIs make it effortless
to ship agents.
*Developers welcome
25K +
25K +
stars on Github
stars on Github



Agent Auth
Let us manage all the headaches of auth for your tools

Tool Observability
Track every tool execution and trigger event in real-time from a single, unified dashboard.

Plug and Play
You can us with any language, any llm and all the frameworks you love (or hate)

Quick to Start —
Effortless to Scale
We can help you scale to billions of tool calls effortlessly

Agent First
Documentation
Our docs and examples are agent first should be easy enough for humans

Agent Auth
Let us manage all the headaches of auth for your tools

Tool Observability
Track every tool execution and trigger event in real-time from a single, unified dashboard.

Agent Auth
Let us manage all the headaches of auth for your tools

Tool Observability
Track every tool execution and trigger event in real-time from a single, unified dashboard.

Plug and Play
You can us with any language, any llm and all the frameworks you love (or hate)

Quick to Start —
Effortless to Scale
We can help you scale to billions of tool calls effortlessly

Agent First
Documentation
Our docs and examples are agent first should be easy enough for humans

Agent Auth
Let us manage all the headaches of auth for your tools

Tool Observability
Track every tool execution and trigger event in real-time from a single, unified dashboard.
Built
for Agents
*
Our easy-to-use APIs make it effortless to ship agents.
*Developers welcome
25K +
stars on Github


Agent Auth
Let us manage all the headaches of auth for your tools

Tool Observability
Track every tool execution and trigger event in real-time from a single, unified dashboard.

Plug and Play
You can us with any language, any llm and all the frameworks you love (or hate)

Quick to Start —
Effortless to Scale
We can help you scale to billions of tool calls effortlessly

Agent First
Documentation
Our docs and examples are agent first should be easy enough for humans

Agent Auth
Let us manage all the headaches of auth for your tools

Tool Observability
Track every tool execution and trigger event in real-time from a single, unified dashboard.

Tool Observability
Track every tool execution and trigger event in real-time from a single, unified dashboard.

Tool Observability
Track every tool execution and trigger event in real-time from a single, unified dashboard.

Plug and Play
You can us with any language, any llm and all the frameworks you love (or hate)

Plug and Play
You can us with any language, any llm and all the frameworks you love (or hate)

Quick to Start —
Effortless to Scale
We can help you scale to billions of tool calls effortlessly

Quick to Start —
Effortless to Scale
We can help you scale to billions of tool calls effortlessly

Agent First
Documentation
Our docs and examples are agent first should be easy enough for humans

Agent First
Documentation
Our docs and examples are agent first should be easy enough for humans

Built-In Auth for
Every Agent
Let us manage all the headaches of auth for your tools

Built-In Auth for
Every Agent
Let us manage all the headaches of auth for your tools






Built a workflow that
used to take 3 services
and 2 meetings.
Built a workflow that
used to take 3 services
and 2 meetings.
Built a workflow that
used to take 3 services
and 2 meetings.
Built a workflow
that used to take 3
services and 2
meetings.
Built a workflow that
used to take 3 services
and 2 meetings.
Power of Thousands of Tools
in the palm of
your agent’s hands.
Agent Auth
We handle the fun stuff like auth
so you can make agents faster.
Evolving Skills
Our skills improve over time as
more agents use them
Composio MCP
Access fully-managed MCP servers
from a single dashboard
Accurate Tool Calls
With tool calls that just work,
your agents perform 30% better.
SOC 2 Type ||
Safe-guard your data
from SKYNET ;)
Power of Thousands of Tools
in the palm of
your agent’s hands.
Agent Auth
We handle the fun stuff like auth
so you can make agents faster.
Evolving Skills
Our skills improve over time as
more agents use them
Composio MCP
Access fully-managed MCP servers
from a single dashboard
Accurate Tool Calls
With tool calls that just work,
your agents perform 30% better.
SOC 2 Type ||
Safe-guard your data
from SKYNET ;)
Thousands of Tools
Access to 3,000+ specific
operations in a single layer
Composio MCP
Access fully managed MCP servers
from a single dashboard
Evolving Skills
Our skills improve over time as
more agents use them
SOC 2 Type II
Enterprise-grade compliance
and secure infrastructure
Accurate Tool Calls
Built-in tool calls help agents
perform 30% better
Agent Auth
We handle the fun stuff like auth
so you can make agents faster

Usage based
Pricing
Usage based
Pricing
Composio is built to help you scale. From
the birth of your first agent to your IPO,
we’re there to grow with you.
Composio is built to help you scale. From
the birth of your first agent to your IPO,
we’re there to grow with you.

Usage based
Pricing
Composio is built to help you scale. From
the birth of your first agent to your IPO,
we’re there to grow with you.

Life rewards action not intelligence
curl -fsSL https://composio.dev/install | bash
curl -fsSL https://composio.dev/install | bash

AI Agents
Connect with over 25 agentic frameworks, allowing AI agents to autonomously plan, coordinate, and execute actions across tools while your agent focuses on planning and execution is handled seamlessly.





LLM
Composio listens to your LLM’s function or tool calls, handles authentication, maps the call to a real-world API, and executes it reliably.


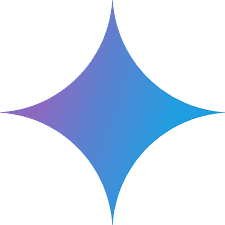

Composio
An all in one seamless layer that lets LLMs and agents reliably interact with tools in the real world.
Authentication
Manage triggers
Set tools and actions
Use multi-agents
Detect bugs in Slack,
auto-log them to
Github and Notion…
Toolkits required



detect bugs in slack
create github issue
log notion entry
update slack thread
time taken →
time taken →
time taken →
time taken →




AI Agent
Lang Graph
Crew AI
Vercel
Lang Chain
Auto Gen
Lang Chain
Lang Graph
AutoGen
Vercel
CrewAI
Tools
LLM
Open AI
Claude
Gemini
Grok
Open AI
Claude
Gemini
Grok

Detect bugs in Slack,
auto-log them to
Github and Notion…
Toolkits required






detect bugs in slack
create github issue
log notion entry
update slack thread
time →
time →
time →
time →
detect bugs in slack
create github issue
log notion entry
update slack thread
time →
time →
time →
time →
AI Agents
Connect with over 25 agentic frameworks, allowing AI agents to autonomously plan, coordinate, and execute actions across tools while your agent focuses on planning and execution is handled seamlessly.










LLM
Composio listens to your LLM’s function or tool calls, handles authentication, maps the call to a real-world API, and executes it reliably.




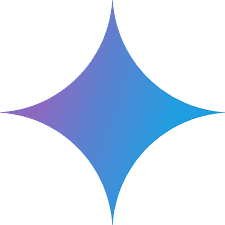
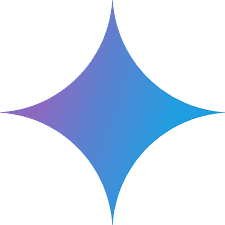


Composio
An all in one seamless layer that lets LLMs and agents reliably interact with tools in the real world.
Authentication
Manage triggers
Set tools and actions
Use multi-agents
Skills that
evolve for
your Agents


More than just integrations, 3000+
tools that can adapt — turning
automation into intuition.


Used by Agents from
Stay updated.
Stay updated.